Welcome back, my aspiring cyberwarriors!
To ascend to the status of cyberwarrior, you must have some basic programming capabilities. In the field of hacking and cybersecurity, this usually means BASH and Python scripting. Python scripting is the most popular among cybersecurity professionals due to its extensive libraries and modules that are useful in cybersecurity (you can use any programming language for cybersecurity but if someone has already created the wheel such as in python, it makes your life much easier). If you examine the tools in Kali, you will find that over 90% are written in Python. As such, I strongly recommend that you develop at least cursory python skills.
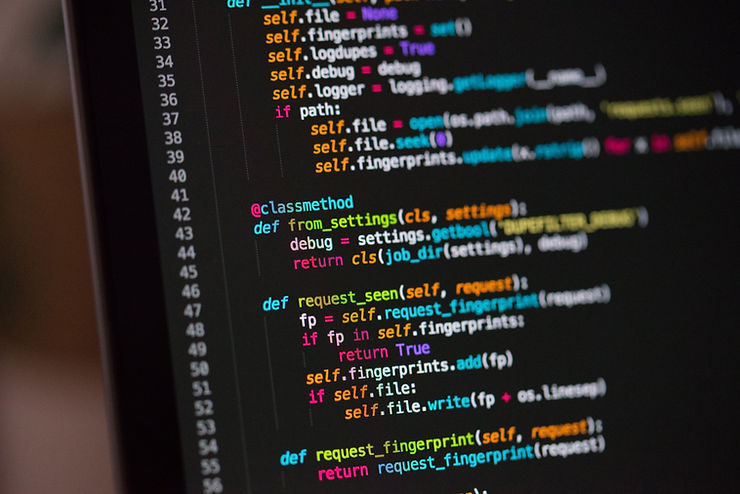
Before starting on your journey in python, I’d like to take a minute or two to familiarize you with some key programming concepts that will help you with any programming language and hopefully will be useful as you embark upon your journey in Python towards becoming a cyberwarrior.
Variables
Variables are just places to store values. Variables enable you to create a storage place and then change (vary) those values as the program runs vs. a static value that never changes.
When writing a program, variables often must be declared before you can use them. Declaring a variable is similar to simply saying “I want this to be a variable”. Once you do that, the system reserves memory for the values that will get placed into the variable.
Generally, you can create (declare) a variable by creating a name with letters of the alphabet, digits, and underscores. These variables can start with an underscore or letters but can not start with a digit.
Types of variables include:
Constant variable – these store data that does not change
Global variable – these variables are accessible throughout (globally) the program
Class variable – these variables are only accessible inside a class (see the section below on OOP).
Instance Variable – these variables are available within the class but outside the method (see OOP below)
Local Variable – these variables are only accessible within the class, method, instance where they are declared (created).
Control structures
In most programs or scripts, there is flow of actions, step 1, then step 2….. At points within that flow a decision must be made, for instance, go right or go left. Control structures are the way we make those decision. They control the flow. That flow usually is dependent upon a value in a variable. For instance, if variable A is less than 10, turn right, if variable A is greater than 10, turn left.
These control structures can be categorized into few general types:
Sequential logic – the program executes in a sequential specific order
Selection logic – these control structures contain a condition that determines which code is executed next.
Iteration logic – a group or block of code it repeated a fixed number of times
Data structures
Data structures enable programs an efficient way of storing and retrieving data. Some common data structures include;
Arrays – arrays store similar elements together such as an ordered list of items. Using contiguous memory locations.
Stacks – these are linear structures that use a last-in, first-out order of executing instructions
Queues – this also is a linear structure but uses a first-in, first out order for executing instructions.
Linked Lists – this is a linear data structure that uses pointers to link elements
Binary Trees – this is a non-linear structure that has nodes that have two different values/directions. These can be used for hierarchical data.
Graphs – these contain nodes and edges connected to each other. These are often used to represent data in complex forms such as maps and social networks.
Object-oriented programming
Before we delve deeper into Python, it’s probably worth taking a few minutes to discuss the concept of object oriented programming (OOP). Python, like most programming languages today (C++, Java, and Ruby, to name a few) adheres to the OOP model. The figure below shows the basic concept behind OOP: the language’s main tool is the object, which has properties in the form of attributes and states, as well as methods that are actions performed by or on the object.
The idea behind OOP-based programming languages is to create objects that act like things in the real world. For example, a car is an object that has properties, such as its wheels, color, size, and engine type; it also has methods, which are the actions the car takes, such as accelerating and locking the doors. From the perspective of natural human language, an object is a noun, a property is an adjective, and a method is generally a verb. Objects are members of a class, which is basically a template for creating objects with shared initial variables, properties, and methods. For instance, say we had a class called cars; our car (a BMW) would be a member of the class of cars. This class would also include other objects/cars, such as Mercedes and Audi

Classes may also have subclasses. Our car class has a BMW subclass, and an object of that subclass might be the model 320i. Each object would have properties (make, model, year, and color) and methods (start, drive, and park), as shown in Figure above
In OOP languages, objects inherit the characteristics of their class, so the BMW 320i would inherit the start, drive, and park methods from class car. These OOP concepts are crucial to understanding how Python and other OOP languages work, as you will see in the scripts in the following sections.
Encapsulation: Encapsulation is the binding of data elements, such as variables and properties and member methods, into one unit.
Abstraction: This enables you to hide specific details about a class and provide only essential information to the outside world. For example, the working mechanism of a web server is unknown to the end-user, who clicks on a button and receives the required information.
Inheritance: This is the ability to create a new class from an existing class. For example, you can create a child class which inherits all the properties of its parent class and has its own set of additional properties and methods.
Polymorphism: Using polymorphism, you can access objects of different types using the same interface where each object provides its own implementation.
Debugging
Debugging is among the most important skills necessary to become a successful coder. This is the process of identifying and resolving errors in your code to produce a functioning script/program.
There are at least three (3) types of errors in code:
Syntax Errors
Logical Errors
Latent Errors
Syntax error
A syntax error occurs when there is an wrong sequence of characters or tokens in the code that doesn’t follow the syntax of a programming language. These may include indentation errors, missing brackets, extra commas, and misspelled commands. Programming tools detect these errors at compile time and display the line number and type of error, along with the error description. You can review the error description and modify the code so that it follows the right syntax.
Logical errors
A logical error occurs when there is a flaw in the algorithm or logic of the code, which either halts the execution of a program or produces a wrong output. You encounter logical errors only during run-time since there are no errors in the code’s syntax. For example, wrongly initializing a variable can cause a logical error in the code.
Latent errors
Latent errors, also known as hidden errors, arise when you use a specific set of data as input to the program. When you forget to account for an outlier or edge case, a program can fail or produce incorrect results. For example, you may have created a program that accepts users’ dates of birth but does not account for characters or negative numbers an end-user may enter incorrectly. Here, the program may either halt its execution or crash.
Programming tools
Integrated development environments or more commonly known as IDE’s are applications that allow programmers to write, compile, and execute code. IDEs provide a central interface that contains tools which facilitate code completion, code compilation, debugging, and syntax highlighting. A good IDE can make your life much easier!
Some IDEs also allow you to add plugins, enabling you to navigate the framework codebase and add your custom classes and features.
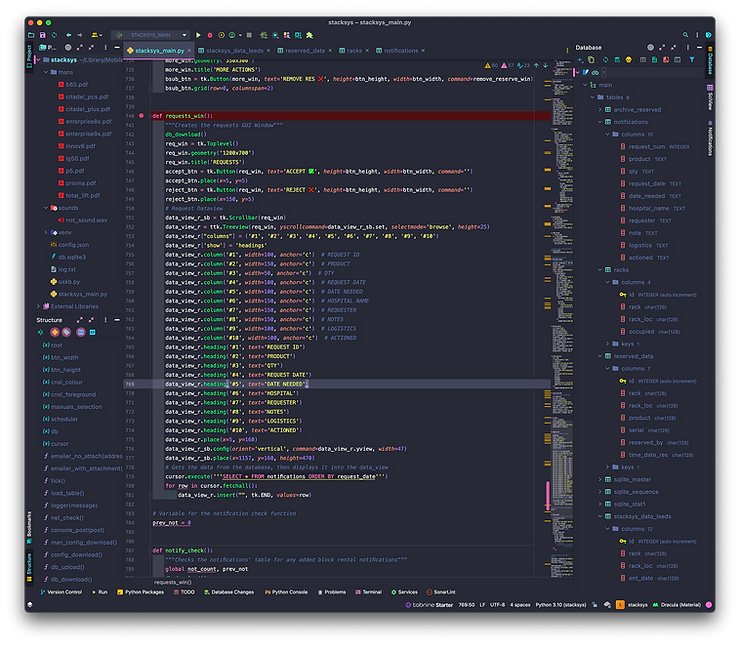
You can use IDEs for:
Writing code: You can use IDEs to write and edit program code to complete a set of tasks.
Compiling code: IDEs compile code by translating human-readable code to a format that is understandable by the computer.
Debugging code: IDEs help you detect errors in a code and make fixes.
Monitoring resources: You can monitor various parameters such as disk space, resource consumption, memory usage, free space on the disk, and cache memory, which help you optimize your code to run faster and more efficiently.
Building automation tools: Some IDEs also provide automated tools for development tasks.
You don’t need an IDE to start coding but they sure can help. Advanced IDE’s such as PyCharm are excellent but also costly. If you are coding in an environment where time is critical, a tool like PyCharm can save you hundreds of hours. Otherwise, simple text editors will work such as vim, gedit, mousepad, and many others are adequate.
If you are working in Kali Linux, there is an excellent free IDE in the Kali repository named Spyder.
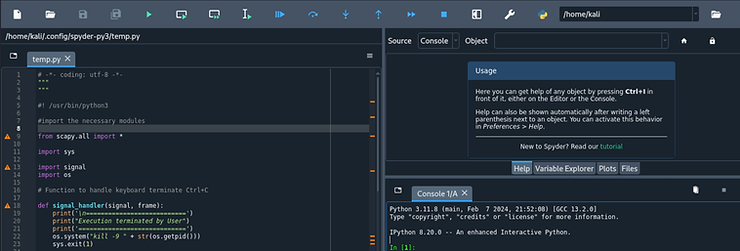
You can download spyder from the Kali repository with the command;
kali > sudo apt install spyder
Summary
Now that you have a general idea of the concepts that are used in programming, the next step is to start coding! If you are in cybersecurity, Python is the language of choice. You can begin learning Python here in our Python Basics for Hackers tutorials or purchase our Python Basics for Hackers videos in our online store.